zz::ImageHdr Class Reference
The ImageHdr class. Image container specifically good for HDR images which uses 32bit float precison. More...
#include <zupply.hpp>
Inheritance diagram for zz::ImageHdr:
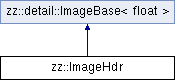
Public Member Functions | |
ImageHdr () | |
ImageHdr Default(empty) constructor. | |
ImageHdr (int rows, int cols, int channels) | |
ImageHdr Constructor given specific size. More... | |
ImageHdr (const char *filename) | |
ImageHdr Constructor from disk image file. More... | |
ImageHdr (const Image &from, float range=1.0f) | |
ImageHdr Constructor from 8-bit image. More... | |
void | load (const char *filename) |
load Load image from disk file, HDR image(*.hdr) supported. More... | |
void | save_hdr (const char *filename) const |
save_hdr Save to HDR image More... | |
Image | to_normal (float range=1.0f) const |
to_normal Convert to 8-bit image(lose precision) More... | |
void | from_normal (const Image &from, float range=1.0f) |
from_normal Convert from an 8-bit image. More... | |
void | resize (Size sz) |
resize Resize image given new size More... | |
void | resize (int width, int height) |
resize Resize image given new height and width More... | |
void | resize (double ratio) |
resize Resize image given ratio to the old size More... | |
![]() | |
ImageBase () | |
ImageBase Default(empty) constructor. | |
ImageBase (int rows, int cols, int channels) | |
ImageBase Constructor with size info. More... | |
ImageBase (const ImageBase &other) | |
ImageBase Copy constructor(shallow copy) More... | |
ImageBase (ImageBase &&other) | |
ImageBase Move constructor. More... | |
void | create (int rows, int cols, int channels) |
create Create storage with specified size More... | |
void | release () |
release Destroy memory storage | |
ImageBase & | operator= (const ImageBase &other) |
operator = Copy operator, again, shallow copy More... | |
ImageBase & | operator= (ImageBase &&other) |
operator = Move operator More... | |
float & | operator() (int row, int col, int channel=0) |
operator () Access pixel element More... | |
const float & | operator() (int row, int col, int channel=0) const |
operator () Access pixel element, immutable version More... | |
operator ImageBase< _Tp2 > () const | |
bool | empty () const |
empty Check empty or not More... | |
int | rows () const |
rows Get number of rows(height, y...) More... | |
int | cols () const |
cols Get number of columns(width, x...) More... | |
int | channels () const |
channels Get number of channels More... | |
float | at (int row, int col, int channel=0) const |
at Access pixel, immutable version. This is guanranteed to be faster than () operator if you don't need to modify data. More... | |
float * | ptr (int offset=0) const |
ptr Data pointer given specifed position. Use with cautious. This is provided for performance consideration. Will not trigger copy-on-write method, so if you change the data, the previous copied images will be affected. More... | |
float * | ptr (int row, int col, int channel=0) const |
ptr Data pointer given specifed position. Use with cautious. This is provided for performance consideration. Will not trigger copy-on-write method, so if you change the data, the previous copied images will be affected. More... | |
void | import (float *data, int rows, int cols, int channels) |
import Import data from raw pointer array. Please make sure the length of array satisfies the size provided. More... | |
void | import (std::vector< float > data, int rows, int cols, int channels) |
import Import data from vector. Please make sure the length of vector satisfies the size provided. More... | |
std::vector< float > | export_raw () const |
std::vector< _Tp2 > & | export_raw (std::vector< _Tp2 > &out) const |
export data to provided vector container. | |
void | crop (int r0, int c0, int r1, int c1) |
crop Crop image given coordinates. More... | |
void | crop (Point p0, Point p1) |
crop Crop image given two points More... | |
void | crop (Rect rect) |
crop Crop image given a rectangle area. More... | |
Additional Inherited Members | |
![]() | |
typedef float | value_type |
![]() | |
void | range_check (long long pos) const |
void | range_check (int row, int col, int channel) const |
void | detach () |
![]() | |
int | rows_ |
int | cols_ |
int | channels_ |
std::shared_ptr< std::vector< float > > | data_ |
Detailed Description
The ImageHdr class. Image container specifically good for HDR images which uses 32bit float precison.
Constructor & Destructor Documentation
|
inline |
ImageHdr Constructor given specific size.
- Parameters
-
rows cols channels
zz::ImageHdr::ImageHdr | ( | const char * | filename | ) |
ImageHdr Constructor from disk image file.
- Parameters
-
filename
zz::ImageHdr::ImageHdr | ( | const Image & | from, |
float | range = 1.0f |
||
) |
ImageHdr Constructor from 8-bit image.
- Parameters
-
from 8-bit image range The range of data stored, normally 1.0 is used. In this case, [0-255] data will be normalized to [0.0-1.0]
Member Function Documentation
void zz::ImageHdr::from_normal | ( | const Image & | from, |
float | range = 1.0f |
||
) |
from_normal Convert from an 8-bit image.
- Parameters
-
from range The range of new stored data, normally 1.0 is used.
void zz::ImageHdr::load | ( | const char * | filename | ) |
load Load image from disk file, HDR image(*.hdr) supported.
- Parameters
-
filename
void zz::ImageHdr::resize | ( | Size | sz | ) |
resize Resize image given new size
- Parameters
-
sz
void zz::ImageHdr::resize | ( | int | width, |
int | height | ||
) |
resize Resize image given new height and width
- Parameters
-
height width
void zz::ImageHdr::resize | ( | double | ratio | ) |
resize Resize image given ratio to the old size
- Parameters
-
ratio
void zz::ImageHdr::save_hdr | ( | const char * | filename | ) | const |
save_hdr Save to HDR image
- Parameters
-
filename
Image zz::ImageHdr::to_normal | ( | float | range = 1.0f | ) | const |
to_normal Convert to 8-bit image(lose precision)
- Parameters
-
range The range of stored data, normally 1.0 is used.
- Returns
- An 8-bit image
The documentation for this class was generated from the following files:
- zupply.hpp
- zupply.cpp