zz::Image Class Reference
The Image class. Image container for 8-bit image manipulation including read/write. Image is based on detail::ImageBase. More...
#include <zupply.hpp>
Inheritance diagram for zz::Image:
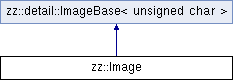
Public Member Functions | |
Image () | |
Image Default(empty) constructor. | |
Image (int rows, int cols, int channels) | |
Image Constructor with specified size. More... | |
Image (const char *filename) | |
Image Constructor from disk image file. More... | |
void | load (const char *filename) |
load Load image from file. More... | |
void | save (const char *filename, int quality=80) const |
save Save image to file. More... | |
void | resize (Size sz) |
resize Resize image given new size More... | |
void | resize (int width, int height) |
resize Resize image given new height and width More... | |
void | resize (double ratio) |
resize Resize image given ratio to the old size More... | |
![]() | |
ImageBase () | |
ImageBase Default(empty) constructor. | |
ImageBase (int rows, int cols, int channels) | |
ImageBase Constructor with size info. More... | |
ImageBase (const ImageBase &other) | |
ImageBase Copy constructor(shallow copy) More... | |
ImageBase (ImageBase &&other) | |
ImageBase Move constructor. More... | |
void | create (int rows, int cols, int channels) |
create Create storage with specified size More... | |
void | release () |
release Destroy memory storage | |
ImageBase & | operator= (const ImageBase &other) |
operator = Copy operator, again, shallow copy More... | |
ImageBase & | operator= (ImageBase &&other) |
operator = Move operator More... | |
unsigned char & | operator() (int row, int col, int channel=0) |
operator () Access pixel element More... | |
const unsigned char & | operator() (int row, int col, int channel=0) const |
operator () Access pixel element, immutable version More... | |
operator ImageBase< _Tp2 > () const | |
bool | empty () const |
empty Check empty or not More... | |
int | rows () const |
rows Get number of rows(height, y...) More... | |
int | cols () const |
cols Get number of columns(width, x...) More... | |
int | channels () const |
channels Get number of channels More... | |
unsigned char | at (int row, int col, int channel=0) const |
at Access pixel, immutable version. This is guanranteed to be faster than () operator if you don't need to modify data. More... | |
unsigned char * | ptr (int offset=0) const |
ptr Data pointer given specifed position. Use with cautious. This is provided for performance consideration. Will not trigger copy-on-write method, so if you change the data, the previous copied images will be affected. More... | |
unsigned char * | ptr (int row, int col, int channel=0) const |
ptr Data pointer given specifed position. Use with cautious. This is provided for performance consideration. Will not trigger copy-on-write method, so if you change the data, the previous copied images will be affected. More... | |
void | import (unsigned char *data, int rows, int cols, int channels) |
import Import data from raw pointer array. Please make sure the length of array satisfies the size provided. More... | |
void | import (std::vector< unsigned char > data, int rows, int cols, int channels) |
import Import data from vector. Please make sure the length of vector satisfies the size provided. More... | |
std::vector< unsigned char > | export_raw () const |
std::vector< _Tp2 > & | export_raw (std::vector< _Tp2 > &out) const |
export data to provided vector container. | |
void | crop (int r0, int c0, int r1, int c1) |
crop Crop image given coordinates. More... | |
void | crop (Point p0, Point p1) |
crop Crop image given two points More... | |
void | crop (Rect rect) |
crop Crop image given a rectangle area. More... | |
Additional Inherited Members | |
![]() | |
typedef unsigned char | value_type |
![]() | |
void | range_check (long long pos) const |
void | range_check (int row, int col, int channel) const |
void | detach () |
![]() | |
int | rows_ |
int | cols_ |
int | channels_ |
std::shared_ptr< std::vector< unsigned char > > | data_ |
Detailed Description
The Image class. Image container for 8-bit image manipulation including read/write. Image is based on detail::ImageBase.
Constructor & Destructor Documentation
|
inline |
Image Constructor with specified size.
- Parameters
-
rows cols channels
zz::Image::Image | ( | const char * | filename | ) |
Image Constructor from disk image file.
- Parameters
-
filename
Member Function Documentation
void zz::Image::load | ( | const char * | filename | ) |
load Load image from file.
- Parameters
-
filename
void zz::Image::resize | ( | Size | sz | ) |
resize Resize image given new size
- Parameters
-
sz
void zz::Image::resize | ( | int | width, |
int | height | ||
) |
resize Resize image given new height and width
- Parameters
-
height width
void zz::Image::resize | ( | double | ratio | ) |
resize Resize image given ratio to the old size
- Parameters
-
ratio
void zz::Image::save | ( | const char * | filename, |
int | quality = 80 |
||
) | const |
save Save image to file.
- Parameters
-
filename quality Save quality(0-100), only applied to JPEG image format.
The documentation for this class was generated from the following files:
- zupply.hpp
- zupply.cpp